How to create a Django project and a Django application
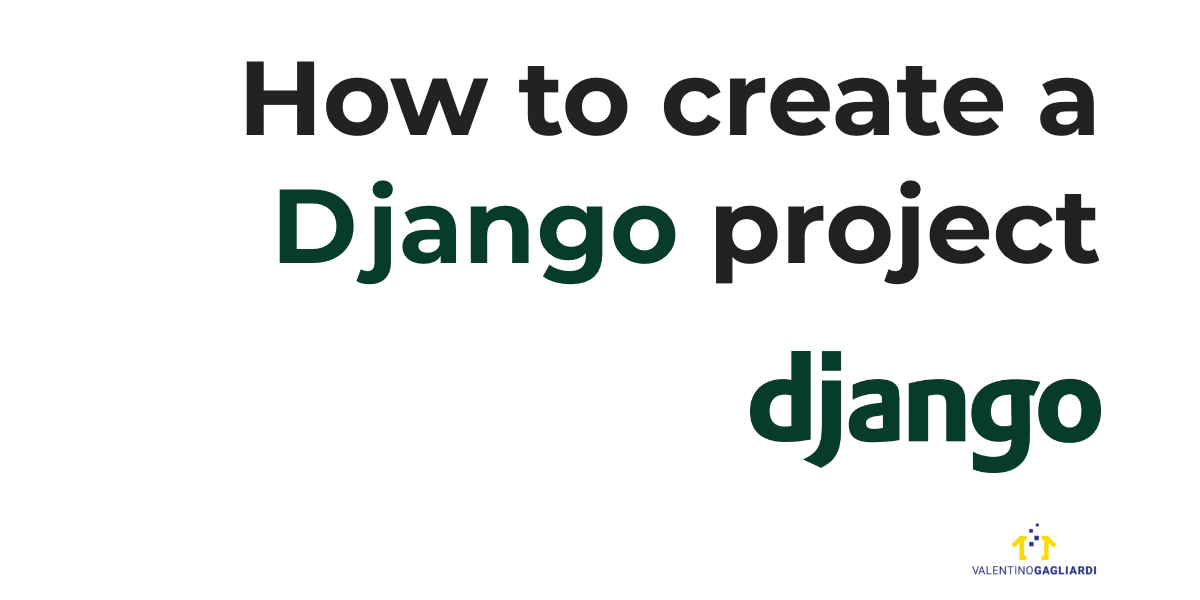
How to create a Django project
To start off create a new folder for the Django project and move into it:
mkdir django-quick-start && cd $_
Here I called django-quick-start
the main folder that will hold the project. Pay attention, this is not the actual Django project, but just its "home". Once inside the new folder create a Python virtual environment and activate it (note, these are two distinct commands):
python3 -m venv venv
source venv/bin/activate
Next up install Django with:
pip install django
When the installer is done you can create a new Django project:
django-admin startproject django_quick_start .
Pay attention again: django_quick_start
now is the actual Django project created inside the django-quick-start
folder. The name of this home folder doesn't matter, but Django projects cannot have dashes in the name. That's the reason for these underscores in django_quick_start
.
Pay also attention to the dot in django-admin startproject django_quick_start .
. With the dot we can avoid additional folder nesting.
How to create a Django application
Every Django project is made of stand alone applications. Each application may have models, views, and custom logic. There is also a great choice of community applications ready to be installed in your project.
Most of the times when you're first starting out all you need is a simple custom app. To create a Django app you can run:
django-admin startapp my_first_app
Where my_first_app
is the name of your app. With the app in place you can start creating things in Django: models, views, and urls, but not before enabling the app in your project's setting. Open up django_quick_start/settings.py
and enable the app in the INSTALLED_APPS list:
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
# enable your app
"my_first_app.apps.MyFirstAppConfig",
]
Next up you can create models in my_first_app/models.py
. Here's a simple model:
from django.db import models
class Customer(models.Model):
first_name = models.CharField(max_length=100)
last_name = models.CharField(max_length=100)
Don't forget to create and apply Django's migrations once the model is in place (note, these are two distinct commands):
python manage.py makemigrations
python manage.py migrate
And now you're ready to run Django!
python manage.py runserver
Where to go from here
In this quick-start tutorial you learned how to create a Django project and a Django application. Now you're ready to build amazing things! Make sure to follow all my Django guides, just scroll below for a list of tutorials and start building!
When you're ready for more serious things grab a cup of tea and learn how to create a Django project from a template.